Ensuring the reliability and availability of your Python applications is crucial for providing a seamless user experience. In this guide, we’ll explore how to enhance your Python application with a Flask health check and integrate it with a Kubernetes livenessProbe
. This combination enables you to not only monitor your application’s health but also allows Kubernetes to automatically handle container restarts based on health status.
As applications grow in complexity, adding a health check becomes a valuable practice. A health check allows you to assess the operational status of your application and take immediate action if any critical components are unavailable. Combining this with a Kubernetes livenessProbe
extends this capability into the realm of container orchestration.
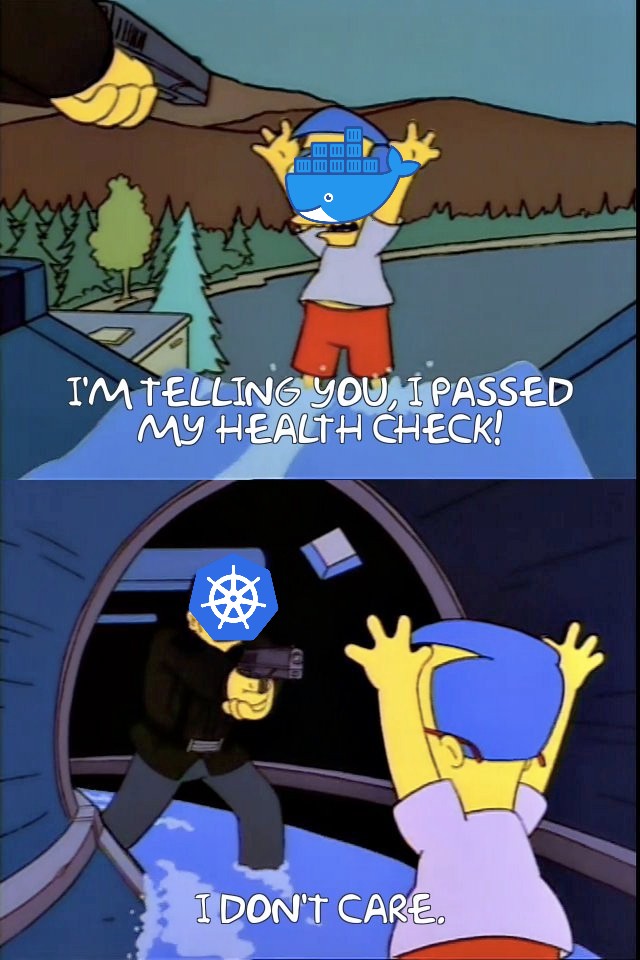
Prerequisites
Before we start, make sure you have the following:
– A Python application that you want to monitor.
– The Flask library installed. If you haven’t installed it yet, you can do so using:
pip install Flask
Integrating Flask Health Check
Step 1: Import Necessary Libraries
from flask import Flask
from threading import Thread
Step 2: Define Flask App and Health Check Logic
app = Flask(__name__)
@app.route('/health')
def health_check():
if all_required_services_are_running():
return 'OK', 200
else:
return 'Service Unavailable', 500
def all_required_services_are_running():
return True
Step 3: Run Flask App in a Separate Thread
def run_flask():
app.run(host='0.0.0.0', port=int(os.environ.get('PORT', 5000)))
if __name__ == '__main__':
# Start the Flask app in a separate thread
flask_thread = Thread(target=run_flask)
flask_thread.start()
Complete code
#!/usr/bin/env python3
from flask import Flask
from threading import Thread
app = Flask(__name__)
@app.route('/health')
def health_check():
# Add your custom health check logic here
if all_required_services_are_running():
return 'OK', 200
else:
return 'Service Unavailable', 500
# Example health check logic, replace it with your actual logic
def all_required_services_are_running():
# Replace this with your logic to check the health of your services
# For example, check if the required processes are running
return True
def run_flask():
app.run(host='0.0.0.0', port=int(os.environ.get('PORT', 5000)))
if __name__ == '__main__':
flask_thread = Thread(target=run_flask)
flask_thread.start()
Testing the Health Check
With the Flask health check integrated into your application, you can now monitor its status by accessing the /health endpoint. A successful health check returns an “OK” response with a 200 status code, indicating that all required services are running. If any services are unavailable, the response will be “Service Unavailable” with a 500 status code.
Kubernetes Liveness Probe
Enhancing Your Deployment with livenessProbe
Now, let’s integrate a Kubernetes livenessProbe
into your deployment to automatically handle container restarts based on health status.
# python-app.yaml
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: python-app
labels:
app: python-app
spec:
replicas: 1
revisionHistoryLimit: 5
selector:
matchLabels:
app: python-app
template:
metadata:
labels:
app: python-app
spec:
containers:
- name: python-app
image: python-app:latest
imagePullPolicy: IfNotPresent
resources:
requests:
memory: "128Mi"
cpu: "0.1"
limits:
cpu: "0.2"
memory: "512Mi"
ports:
- containerPort: 80
livenessProbe:
httpGet:
path: /health
port: 5000
initialDelaySeconds: 3
periodSeconds: 60
now let’s install it:
kubectl apply -f python-app.yaml
# The liveness probe helps Kubernetes determine if your container is healthy and responsive
# It periodically sends an HTTP request to the specified path and port and checks for a successful response
# If the health check fails, Kubernetes may restart or take other actions based on your container orchestration policies
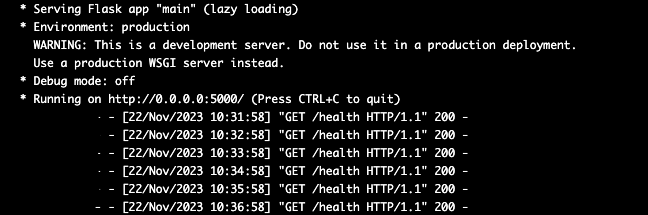
In this Kubernetes manifest:
- We’ve added the
livenessProbe
section to the container specification. - The
livenessProbe
sends periodic HTTP requests to the health check endpoint (/health
) to ensure the container is healthy. - Kubernetes will automatically restart the container if the health check fails, helping to maintain the overall health of your application.
Testing the Setup
With the Flask health check and Kubernetes livenessProbe
integrated into your application and deployment, you can now monitor your application’s status and ensure that Kubernetes takes appropriate actions in case of any issues.
Combining a Flask health check with a Kubernetes livenessProbe
is a powerful way to ensure the continuous health and reliability of your Python application. This setup allows you to proactively monitor your application and automatically handle container restarts based on health status, contributing to a seamless and resilient user experience.
Feel free to customize the health check logic and Kubernetes configuration based on your specific application requirements.
Happy coding!