Last updated on November 22, 2023
In the fast-paced world of web development, streamlining the deployment process is crucial. Docker has emerged as a powerful tool for containerization, allowing developers to encapsulate their applications and dependencies into portable containers. In this guide, we’ll walk through the process of Dockerizing a React app for both development and production environments.
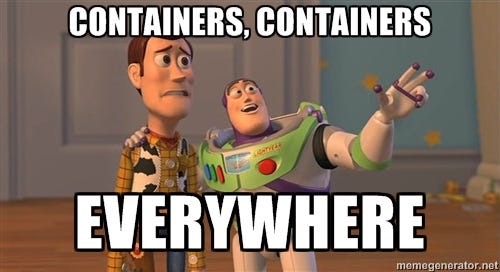
Prerequisites:
Before diving into Dockerizing your React app, ensure you have the following tools installed on your machine:
– Node.js and npm
– Docker
– React
Step 1: Set Up Your React App:
If you don’t have a React app yet, create one using Create React App or your preferred method:
npx create-react-app my-react-app
cd my-react-app
Step 2: Dockerfile for Development:
Add a .dockerignore
file
node_modules
npm-debug.log
build
.git
*.md
.gitignore
Create a Dockerfile.dev in the root of your project for development. This file will use a lightweight Node.js image and copy your app files into the container:
# Use an official Node.js runtime as a base image
FROM node:14-alpine
# Set the working directory inside the container
WORKDIR /app
# Copy package.json and package-lock.json to the working directory
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy the local project files to the working directory
COPY . .
# Expose the port the app runs on
EXPOSE 3000
# Start the app
CMD ["npm", "start"]
Step 3: Docker Compose for Development:
Create a docker-compose.yml file to simplify container orchestration for development:
version: '3'
services:
web:
build:
context: .
dockerfile: Dockerfile.dev
ports:
- "3000:3000"
volumes:
- /app/node_modules
- .:/app
Run your app using:
docker-compose up
docker-compose down
Step 4: Dockerfile for Production:
Configure nginx
Create a nginx.conf
with the below content. This will help handle URI changes during routing.
server {
listen 80;
location / {
root /usr/share/nginx/html/;
include /etc/nginx/mime.types;
try_files $uri $uri/ /index.html;
}
}
Create a Dockerfile for production, leveraging a multi-stage build for a smaller final image:
FROM node:14-alpine AS builder
ENV NODE_ENV production
WORKDIR /app
COPY ./package*.json ./
RUN npm install
COPY . .
RUN npm run build
FROM nginx
COPY --from=builder /app/build /usr/share/nginx/html
COPY nginx.conf /etc/nginx/conf.d/default.conf
EXPOSE 80 443
CMD [ "nginx", "-g", "daemon off;" ]
Step 5: Build and Run for Production:
Build and run your production-ready Docker container:
docker build -t my-react-app .
docker run -p 8080:80 my-react-app
Visit http://localhost:8080 in your browser to see your React app running in a production-ready Docker container.
Dockerizing your React app for both development and production environments provides consistency, portability, and scalability. With this guide, you should be able to efficiently manage your React app’s containerization, ensuring a smoother development workflow and a more straightforward production deployment process.