To make manual approval in Jenkins pipeline, you can use the input step. Here’s an example:
pipeline {
agent any
stages {
stage('Build') {
steps {
// build your code here
}
}
stage('Approval') {
steps {
script {
def deploymentDelay = input id: 'Deploy', message: 'Deploy to production?', submitter: 'admin', parameters: [choice(choices: ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '10', '11', '12', '13', '14', '15', '16', '17', '18', '19', '20', '21', '22', '23', '24'], description: 'Hours to delay deployment?', name: 'deploymentDelay')]
sleep time: deploymentDelay.toInteger(), unit: 'HOURS'
}
}
}
stage('Deploy') {
steps {
// deploy your code here after manual approve
}
}
}
}
stage(‘Approval’) lets you manually approve, decline or postpone for 24 hours
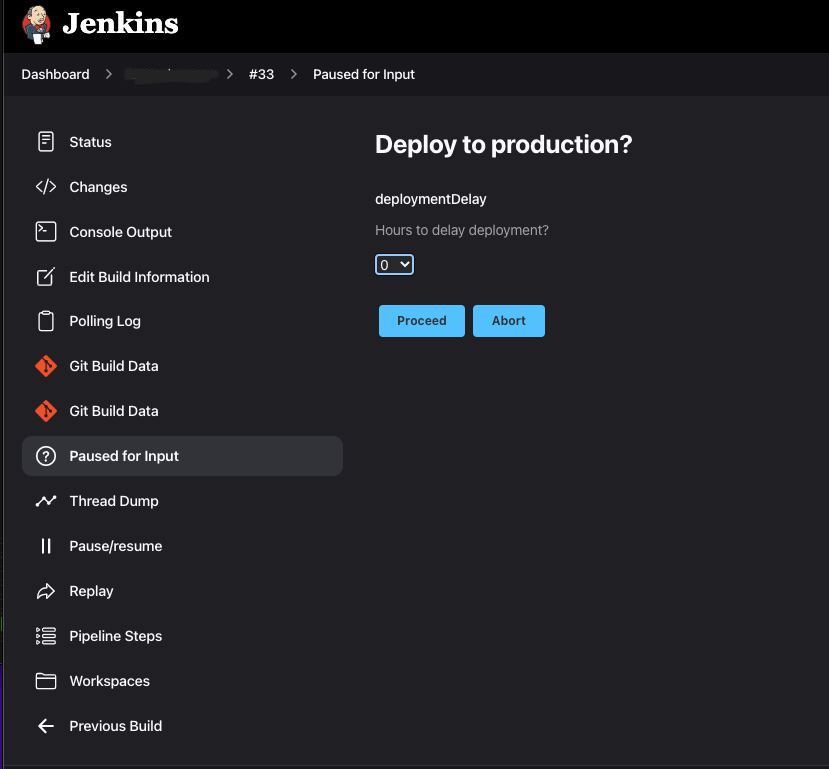
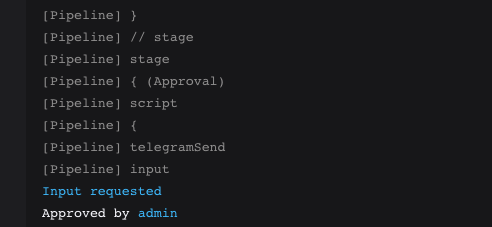
Let’s make it even interesting and add telegram notifications for Approval stage.
First we need to create telegram bot in @botfather , then we install Telegram Bot Plugin for Jenkins here.
Setup
Create a Telegram bot
- Find BotFather in Telegram (@BotFather)
- Send /newbot command
- Enter bot name and bot username
Jenkins Global configuration
- Open the Jenkins global config
- Paste your bot name and username to according textfields
- In filed Usernames fill names of users who can get Jenkins messages (separated by spaces)
- Save
Subscribe for Jenkins messages
- In telegram find your bot and send /start command
- Send */sub *command
Configuration
Manage your job
1. Add build-step (or post build-step.
2. Fill the message (you can use environment variables and simple Markdown)
3. Save your job
Then we need to configure our pipeline.
pipeline {
agent any
stages {
stage('Build') {
steps {
// build your code here
}
}
stage('Approval') {
steps {
script {
telegramSend "⌛️ *$env.JOB_NAME* #$env.BUILD_NUMBER - *Deploy to PROD?* \n$env.BUILD_URL"
def deploymentDelay = input id: 'Deploy', message: 'Deploy to production?', submitter: 'admin', parameters: [choice(choices: ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '10', '11', '12', '13', '14', '15', '16', '17', '18', '19', '20', '21', '22', '23', '24'], description: 'Hours to delay deployment?', name: 'deploymentDelay')]
sleep time: deploymentDelay.toInteger(), unit: 'HOURS'
}
}
}
stage('Deploy') {
steps {
// deploy your code here after manual approve
}
}
}
post {
success {
telegramSend "✅ *$env.JOB_NAME* #$env.BUILD_NUMBER - *SUCCESS* \n$env.BUILD_URL \n Deployed to ${AGENT_LABEL}"
}
failure {
telegramSend "❌ *$env.JOB_NAME* #$env.BUILD_NUMBER - *FAILED*, \nplease check $env.BUILD_URL"
}
aborted {
telegramSend "⚫️ *$env.JOB_NAME* #$env.BUILD_NUMBER - *ABORTED*, \nplease check $env.BUILD_URL"
}
}
}
The result looks like this
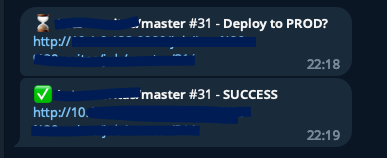
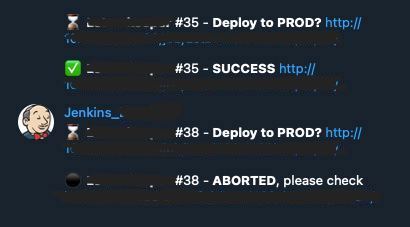