Last updated on February 6, 2024
In today’s fast-paced digital landscape, organizations are increasingly relying on containerization to efficiently deploy and manage their applications. Kubernetes, an open-source container orchestration platform, has emerged as the de facto standard for automating the deployment, scaling, and management of containerized applications. One of the key aspects of Kubernetes is its diverse array of deployment strategies that allow developers to implement various rollout and scaling mechanisms. In this blog post, we will explore the different Kubernetes deployment strategies and how they can empower you to deliver applications seamlessly.
1. Rolling Updates:
The Rolling Updates strategy is one of the most widely used deployment techniques in Kubernetes. It ensures that new versions of applications are gradually rolled out while the previous versions are phased out. This strategy minimizes downtime and allows for easy rollback if any issues arise during the deployment process. By defining parameters such as the number of replicas, the maximum surge (extra replicas allowed during the update), and the maximum unavailable (how many pods can be unavailable), developers can carefully control the update process.
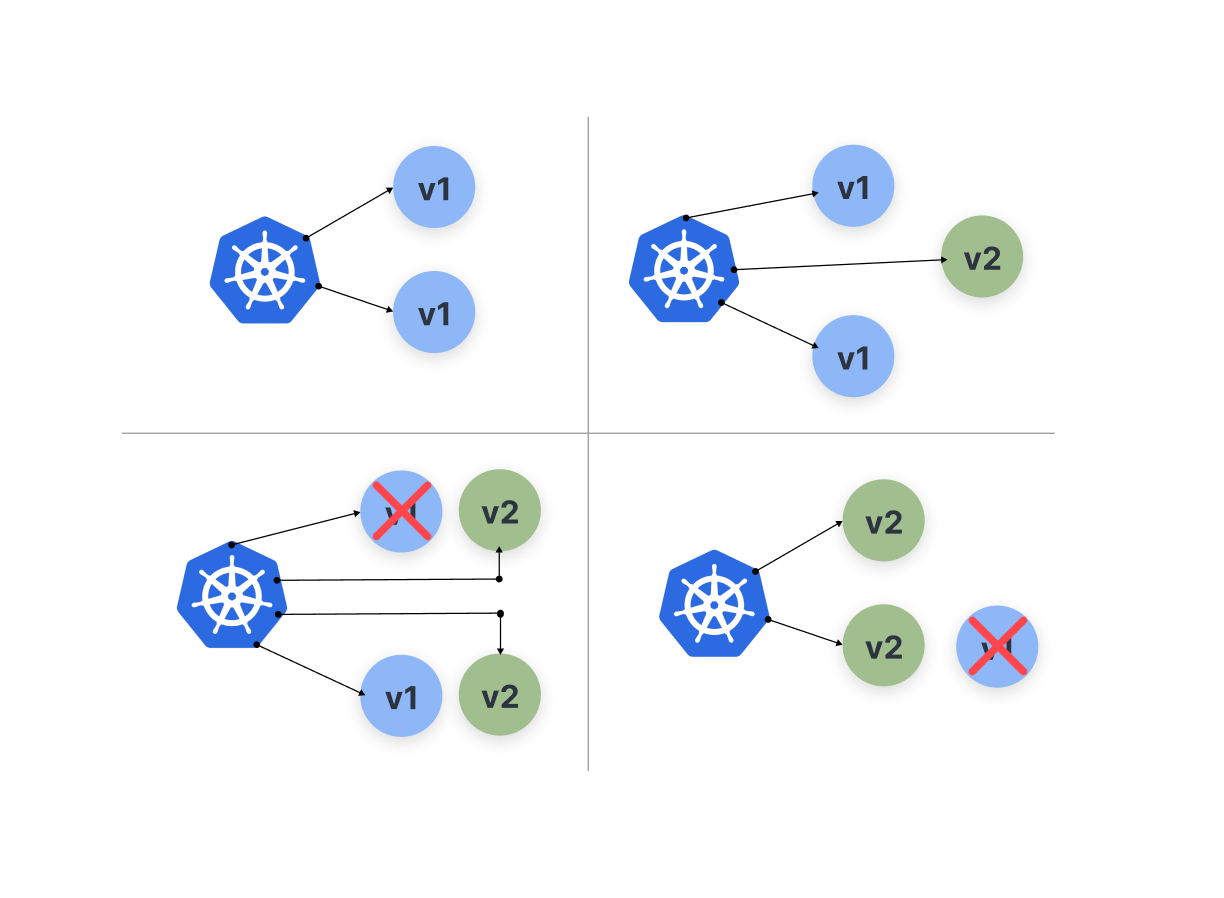
Here’s an example of a YAML configuration for the Rolling update strategy:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: my-app:latest
ports:
- containerPort: 8080
2. Blue-Green Deployments:
Blue-Green Deployments provide a way to minimize the impact of new releases on the production environment. In this strategy, two identical environments (blue and green) are maintained, with only one actively serving traffic at a time. The new version is deployed to the inactive environment, ensuring that it undergoes thorough testing before being switched to the active environment. This approach allows for instant rollback in case of issues and provides a seamless transition between versions with zero downtime.
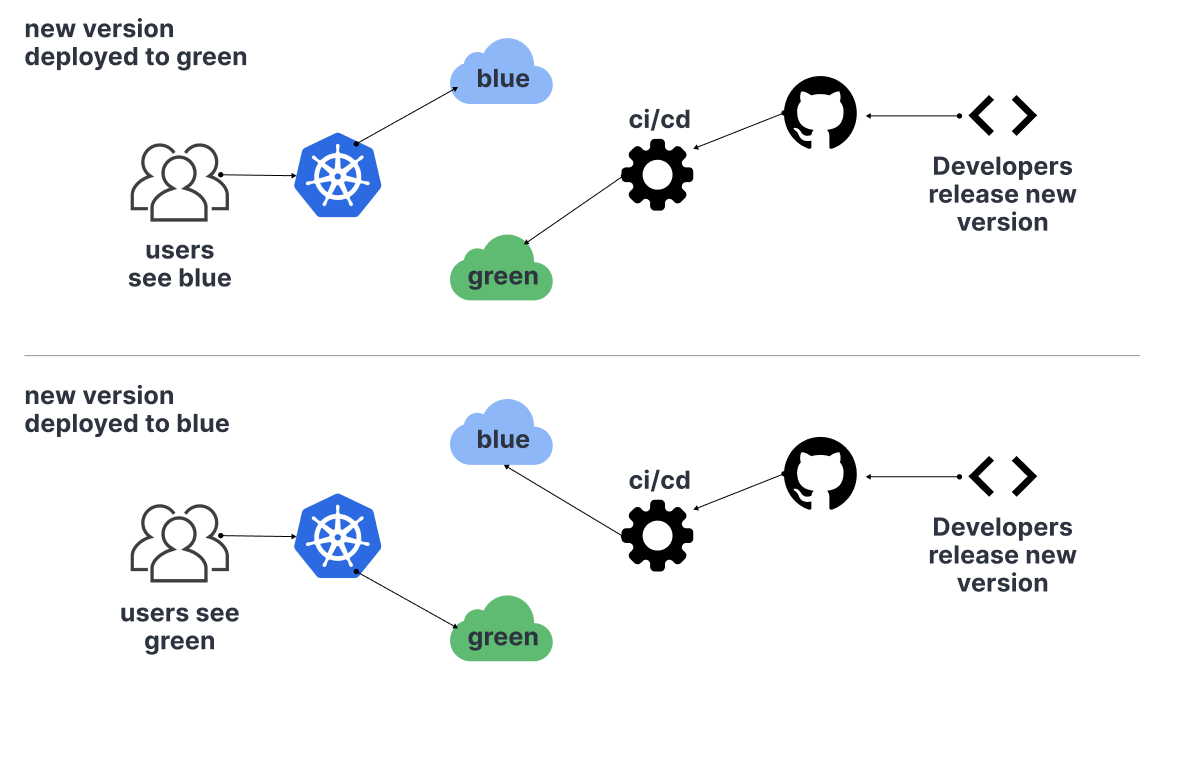
Here’s an example of a YAML configuration for Blue-Green strategy:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-blue
spec:
replicas: 3
selector:
matchLabels:
app: my-app-blue
template:
metadata:
labels:
app: my-app-blue
spec:
containers:
- name: my-app
image: my-app:blue
ports:
- containerPort: 8080
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-green
spec:
replicas: 0
selector:
matchLabels:
app: my-app-green
template:
metadata:
labels:
app: my-app-green
spec:
containers:
- name: my-app
image: my-app:green
ports:
- containerPort: 8080
3. Canary Deployments:
Canary Deployments enable organizations to gradually test new versions with a subset of users or traffic before fully rolling out the changes. By diverting a small percentage of traffic to the new version, developers can closely monitor its performance and gather valuable feedback. Kubernetes offers features like traffic splitting and weighted routing that allow for precise control over the distribution of traffic between different versions. Canary deployments provide an extra layer of confidence, as any issues can be detected and addressed before impacting the entire user base.
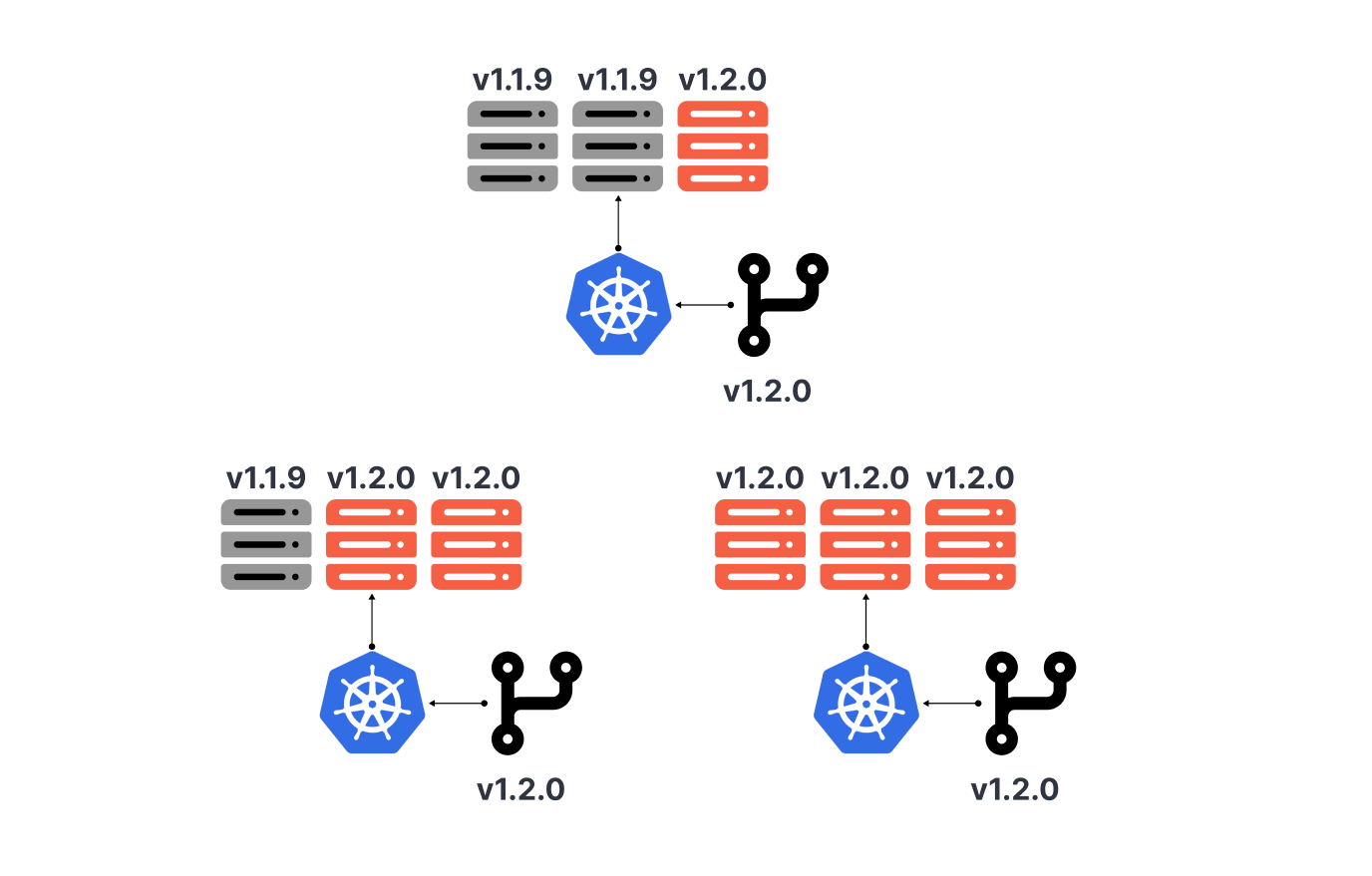
Here’s an example of a YAML configuration for the Canary strategy:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 3
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: my-app:latest
ports:
- containerPort: 8080
---
apiVersion: networking.k8s.io/v1beta1
kind: Ingress
metadata:
name: my-app-ingress
spec:
rules:
- http:
paths:
- path: /
backend:
serviceName: my-app
servicePort: 8080
---
- path: /canary
backend:
serviceName: my-app-canary
servicePort: 8080
4. A/B Testing:
A/B Testing is a deployment strategy that focuses on comparing two versions of an application to determine which one performs better based on predefined metrics. By splitting traffic between the two versions, developers can gather statistical data on user behavior and preferences. Kubernetes allows for easy A/B testing by leveraging features like service mesh and ingress controllers. This strategy is particularly useful for iterative improvements and optimizing user experience.
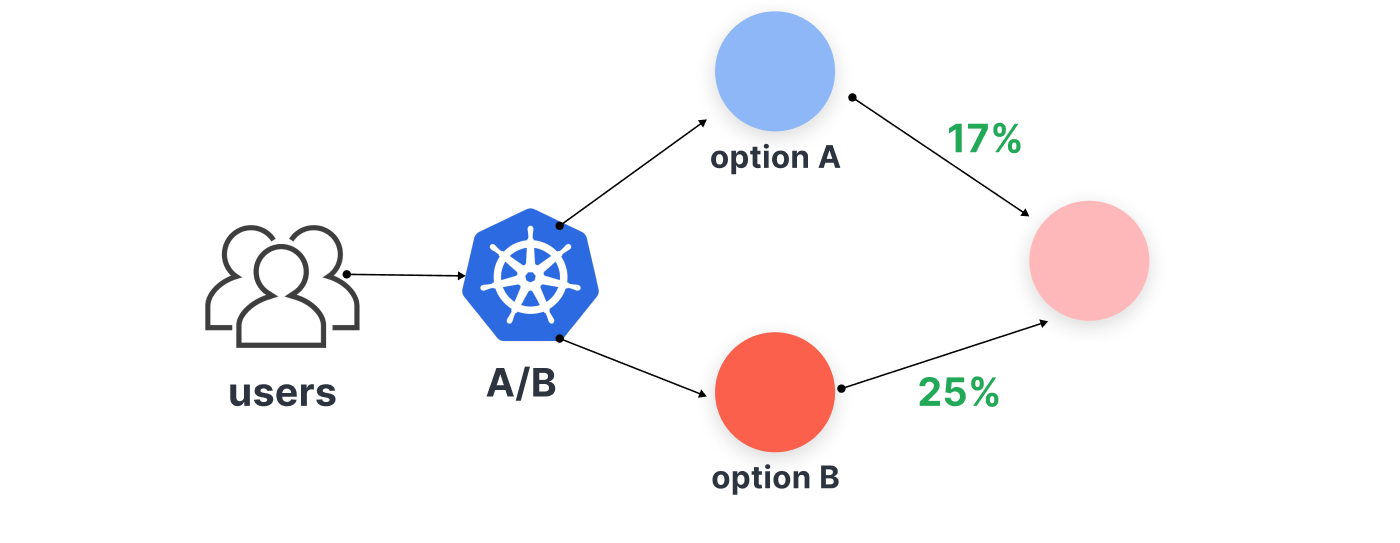
Here’s an example of a YAML configuration for A/B strategy:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-a
spec:
replicas: 3
selector:
matchLabels:
app: my-app-a
template:
metadata:
labels:
app: my-app-a
spec:
containers:
- name: my-app
image: my-app:a
ports:
- containerPort: 8080
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-b
spec:
replicas: 3
selector:
matchLabels:
app: my-app-b
template:
metadata:
labels:
app: my-app-b
spec:
containers:
- name: my-app
image: my-app:b
ports:
- containerPort: 8080
---
apiVersion: networking.k8s.io/v1beta1
kind: Ingress
metadata:
name: my-app-ingress
spec:
rules:
- http:
paths:
- path: /
backend:
serviceName: my-app-a
servicePort: 8080
---
- path: /b
backend:
serviceName: my-app-b
servicePort: 8080
5. Blue-Green with Testing in Production:
While traditional blue-green deployments involve testing in a separate environment, this strategy takes it a step further by performing testing directly in the production environment. By leveraging Kubernetes’ capabilities to create isolated namespaces or dedicated clusters, developers can safely test new features without affecting the main user base. This approach allows for quicker feedback and reduces the time required for feature validation.
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-blue
spec:
replicas: 3
selector:
matchLabels:
app: my-app-blue
template:
metadata:
labels:
app: my-app-blue
spec:
containers:
- name: my-app
image: my-app:blue
ports:
- containerPort: 8080
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app-green
spec:
replicas: 0
selector:
matchLabels:
app: my-app-green
template:
metadata:
labels:
app: my-app-green
spec:
containers:
- name: my-app
image: my-app:green
ports:
- containerPort: 8080
---
apiVersion: networking.k8s.io/v1beta1
kind: Ingress
metadata:
name: my-app-ingress
spec:
rules:
- http:
paths:
- path: /
backend:
serviceName: my-app-blue
servicePort: 8080
---
- path: /test
backend:
serviceName: my-app-green
servicePort: 8080
6. Recreate Strategy:
The Recreate strategy is a straightforward deployment approach that involves terminating all existing pods before creating new ones with the updated version. Unlike the Rolling Updates strategy, which gradually replaces pods, the Recreate strategy ensures a clean break between versions. This approach is suitable when you want to quickly replace the entire application with a new version without considering high availability or minimizing downtime.
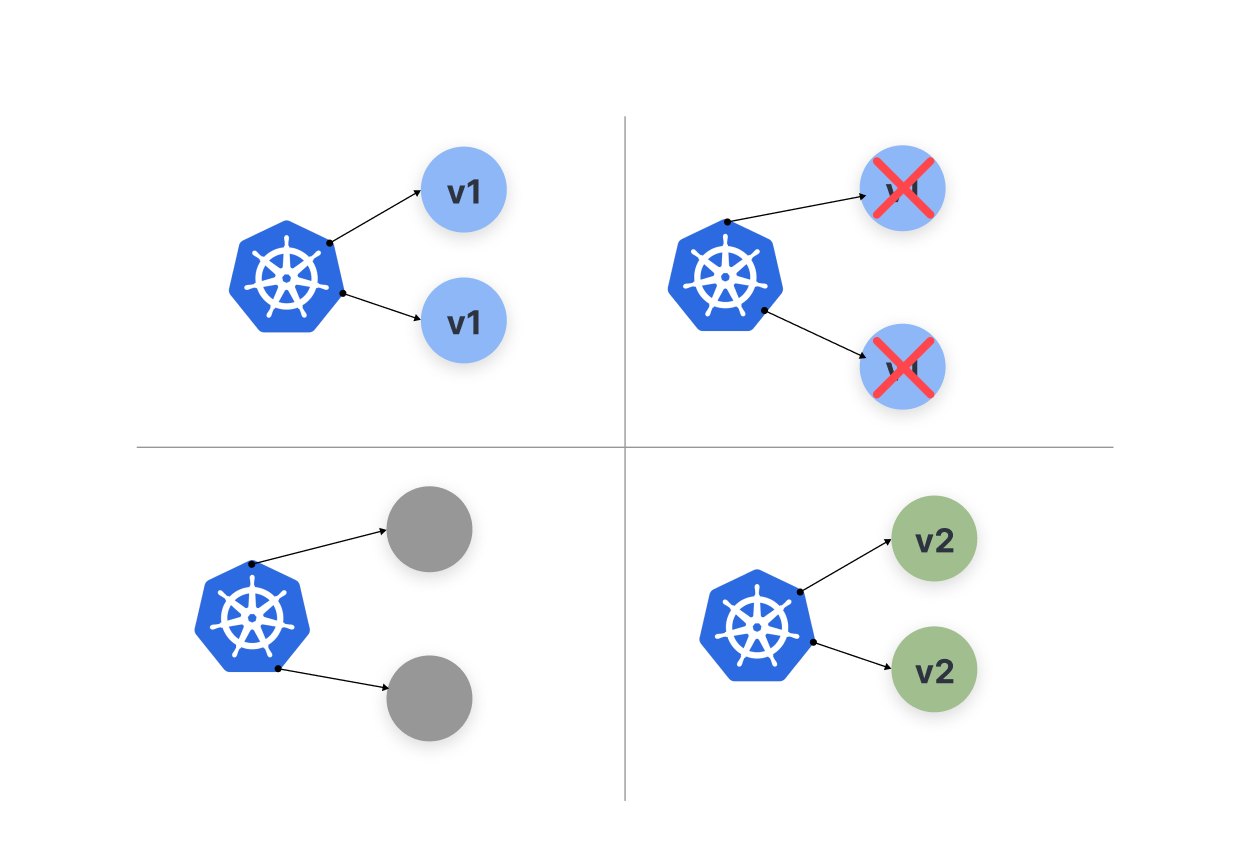
Here’s an example of a YAML configuration for the Recreate strategy:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 3
strategy:
type: Recreate
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: my-app:latest
ports:
- containerPort: 8080
Conclusion:
Kubernetes provides a rich set of deployment strategies that empower developers to deliver applications seamlessly, with reduced downtime and increased reliability. From rolling updates to canary deployments, each strategy offers unique benefits depending on specific use cases and organizational needs. By understanding and leveraging these deployment strategies effectively, organizations can optimize their application delivery, improve user experience, and stay ahead in the rapidly evolving digital landscape.
Remember, choosing the right deployment strategy depends on factors such as the application’s criticality, user impact tolerance, and release requirements. Experimentation, monitoring, and continuous improvement are key to mastering Kubernetes deployment strategies and achieving successful application deployments.